40 Hours
Prior programming experience with C.
You will learn how to;
- Writing procedural programs using C++
- Using private, public and protected keywords to control access to class members
- Defining a class in C++
- Writing constructors and destructors
- Writing classes with const and static class members
- Overloading operators
- Implementing polymorphic methods in programs
- Writing programs using file I/O and string streams
- Using manipulators and stream flags to format output
- Using the keyword template to write generic functions and classes
- Writing programs that use generic classes and functions
- Writing programs that use algorithms and containers of the Standard Library
- Using algorithms and containers of the Standard Library to manipulate string data
- Using try() blocks to trap exceptions
- Using catch() blocks to handle exceptions
- Defining exceptions and using throw to trigger them
Upon successful completion of the training, you will be issued with; C++ certification.
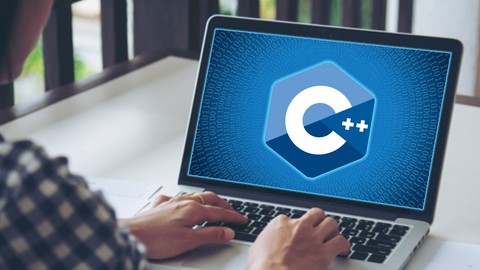